你有没有想过,用代码也能打造出翱翔天际的小飞机呢?没错,就是那种在屏幕上飞来飞去的酷炫小飞机!今天,我就要带你一起探索,怎样用代码让这个小家伙在屏幕上展翅高飞。
一、选择你的舞台:图形库的选择
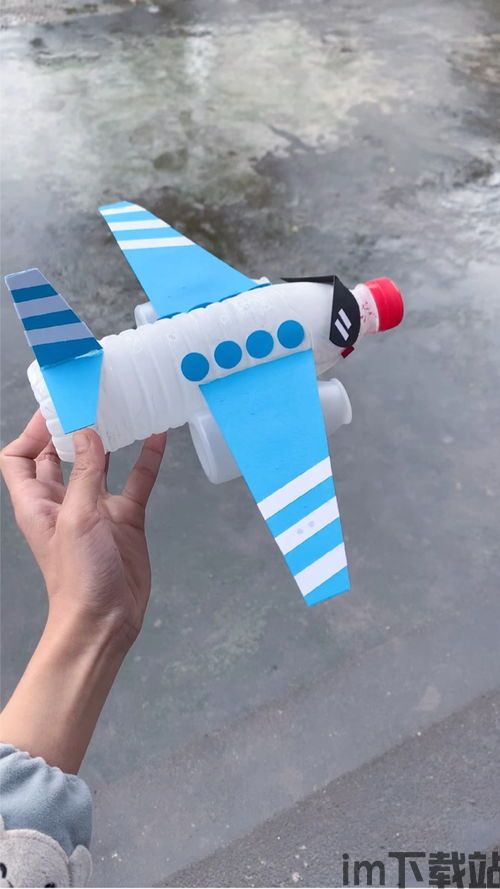
首先,你得有个舞台,也就是图形库。在Java的世界里,Swing和JavaFX都是不错的选择。Swing比较经典,JavaFX则更现代一些。如果你是C语言爱好者,那么SDL或Allegro这样的图形库也能满足你的需求。
二、搭建你的小飞机:面向对象编程
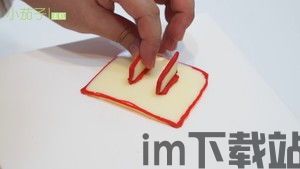
接下来,我们要用面向对象编程的思想,给小飞机设计一个完美的“身体”。在Java中,我们可以创建一个Plane类,里面包含小飞机的位置、速度、图片等属性,以及移动、射击等行为。
```java
public class Plane {
private int x, y;
private int speed;
private Image image;
public Plane(int x, int y, int speed, Image image) {
this.x = x;
this.y = y;
this.speed = speed;
this.image = image;
}
public void move() {
// 实现小飞机的移动逻辑
}
public void shoot() {
// 实现小飞机的射击逻辑
}
三、绘制你的舞台:图形用户界面(GUI)
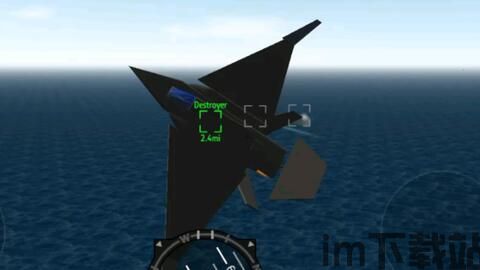
有了小飞机,我们还需要一个舞台来展示它。在Java中,我们可以使用JFrame作为游戏主窗口,JPanel作为绘制游戏画面的区域。我们就可以用Graphics类来绘制小飞机了。
```java
public class GamePanel extends JPanel {
private Plane plane;
public GamePanel(Plane plane) {
this.plane = plane;
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
g.drawImage(plane.getImage(), plane.getX(), plane.getY(), this);
}
四、让小飞机动起来:事件处理
为了让小飞机动起来,我们需要处理用户的输入。在Java中,我们可以使用KeyListener来监听键盘按键。当用户按下空格键时,小飞机就可以发射子弹了。
```java
public class GameFrame extends JFrame {
private GamePanel gamePanel;
public GameFrame() {
gamePanel = new GamePanel(new Plane(100, 100, 5, new Image(\plane.png\)));
this.add(gamePanel);
this.addKeyListener(new KeyAdapter() {
@Override
public void keyPressed(KeyEvent e) {
if (e.getKeyCode() == KeyEvent.VK_SPACE) {
gamePanel.getPlane().shoot();
}
}
});
this.setSize(800, 600);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setVisible(true);
}
public static void main(String[] args) {
new GameFrame();
}
五、让小飞机飞得更远:线程与并发
为了让小飞机在屏幕上飞得更加流畅,我们可以使用多线程。在Java中,我们可以创建一个新的线程来处理小飞机的移动逻辑。
```java
public class PlaneMovementThread extends Thread {
private Plane plane;
public PlaneMovementThread(Plane plane) {
this.plane = plane;
}
@Override
public void run() {
while (true) {
plane.move();
try {
Thread.sleep(10);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
六、碰撞检测:让游戏更有趣
为了让游戏更有趣,我们需要实现碰撞检测。当小飞机与子弹或敌机碰撞时,游戏就结束了。
```java
public class CollisionDetector {
public static boolean isCollided(Plane plane, Bullet bullet) {
// 实现碰撞检测逻辑
}
通过以上步骤,你就可以用代码打造出一个属于自己的小飞机游戏了。快来试试吧,让你的创意在屏幕上翱翔吧!